How to get started with Reown AppKit on any EVM chain
Learn how to use Reown AppKit to enable wallet connections and interact with any EVM network. With AppKit, you can provide seamless wallet connections, including email and social logins, on-ramp functionality, smart accounts, one-click authentication, and wallet notifications, all designed to deliver an exceptional user experience.
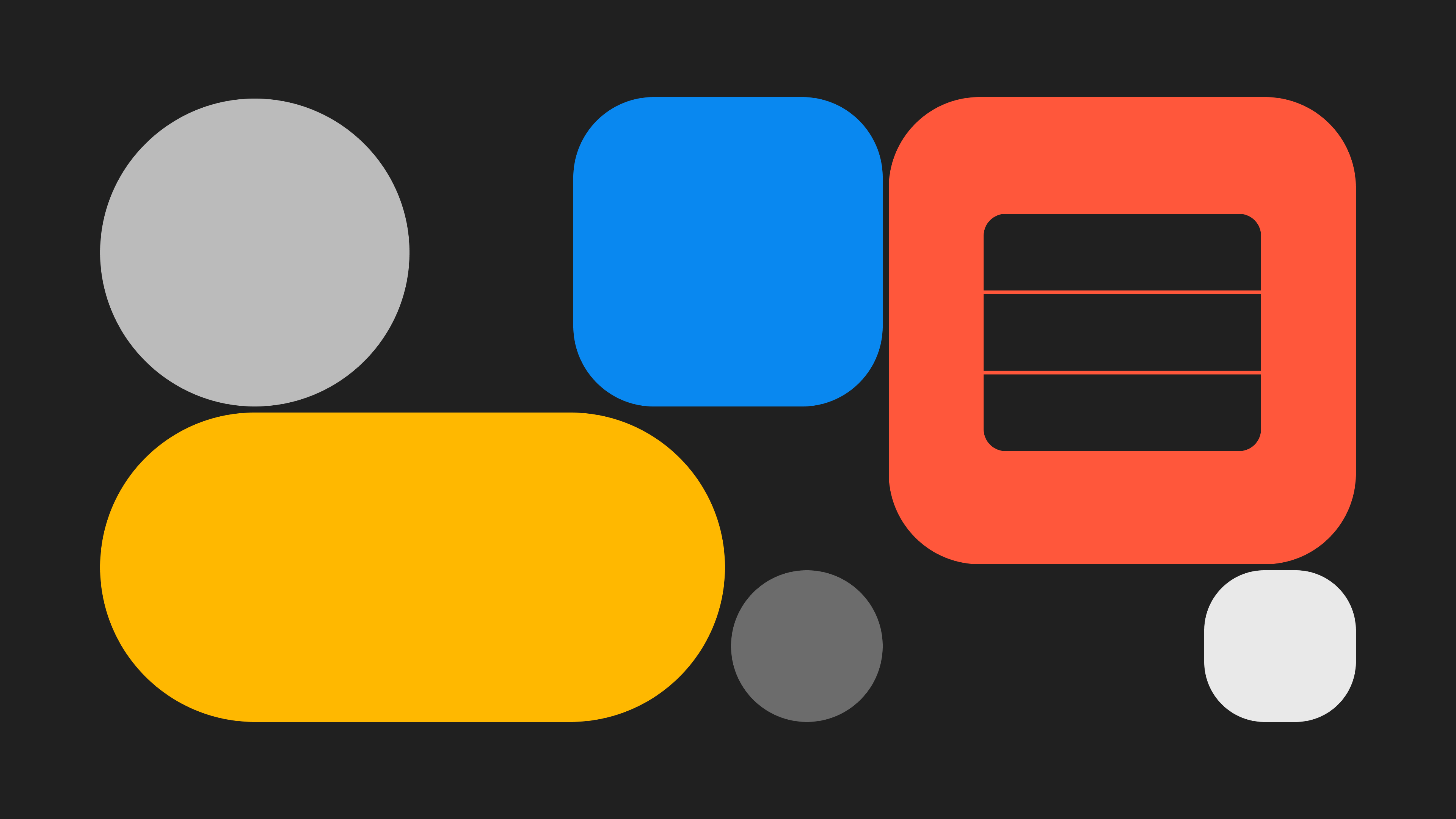
In this tutorial, you will learn how to:
1. Set up Reown AppKit.
2. Configure a wallet connection modal and enable interactions with any EVM network.
This guide takes approximately 10 minutes to complete.
Let’s get started!
Setup
In this section, you'll learn how to set up the development environment to use AppKit with EVM.
For this tutorial, we'll be using Next.js, though you can use any other framework compatible with AppKit.
AppKit is available on eight frameworks, including React, Next.js, Vue, JavaScript, React Native, Flutter, Android, iOS, and Unity.
Now, let’s create a Next app. In order to do so, please run the command given below:
npx create-next-app@latest appkit-example
The above command creates a Next app and sets the name of the Next app as “appkit-example”.
Install AppKit
Now, we need to install AppKit and other dependencies that we need for our app to function as expected. For this tutorial, we will be using “wagmi” as our preferred Ethereum library. However, you can also use Ethers.
npm install @reown/appkit @reown/appkit-adapter-wagmi wagmi viem @tanstack/react-query
You can also use other package managers such as yarn, bun, pnpm, etc.
Create a new project on Reown Cloud
Now, we need to get a project Id from Reown Cloud that we will use to set up AppKit with Wagmi config. Navigate to cloud.reown.com and sign in. If you have not created an account yet, please do so before we proceed.
After you have logged in, please navigate to the “Projects” section of the Cloud and click on “Create Project”.
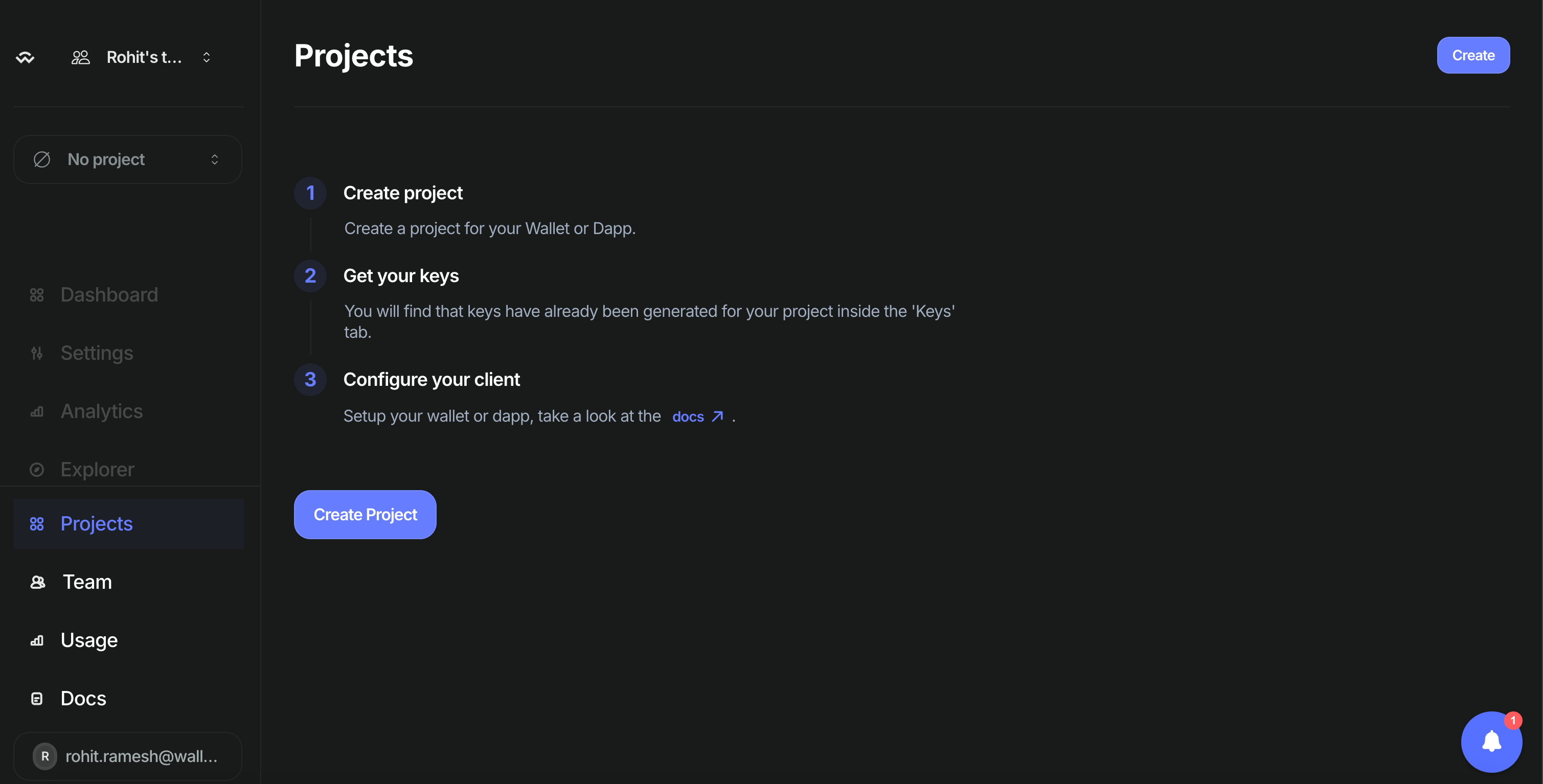
Now, enter the name for your project and click on “Continue”.
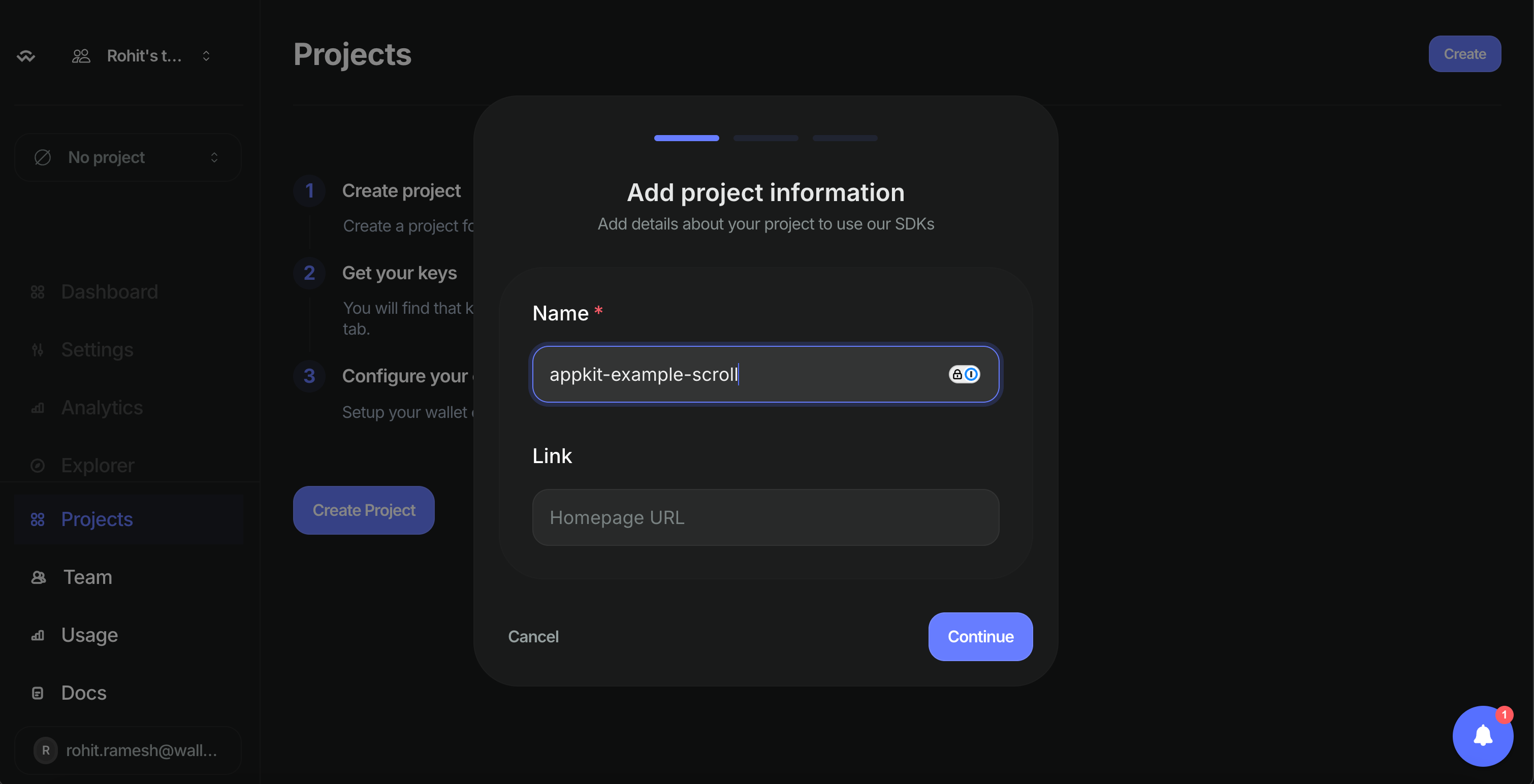
Select the product as “AppKit” and click on “Continue”.
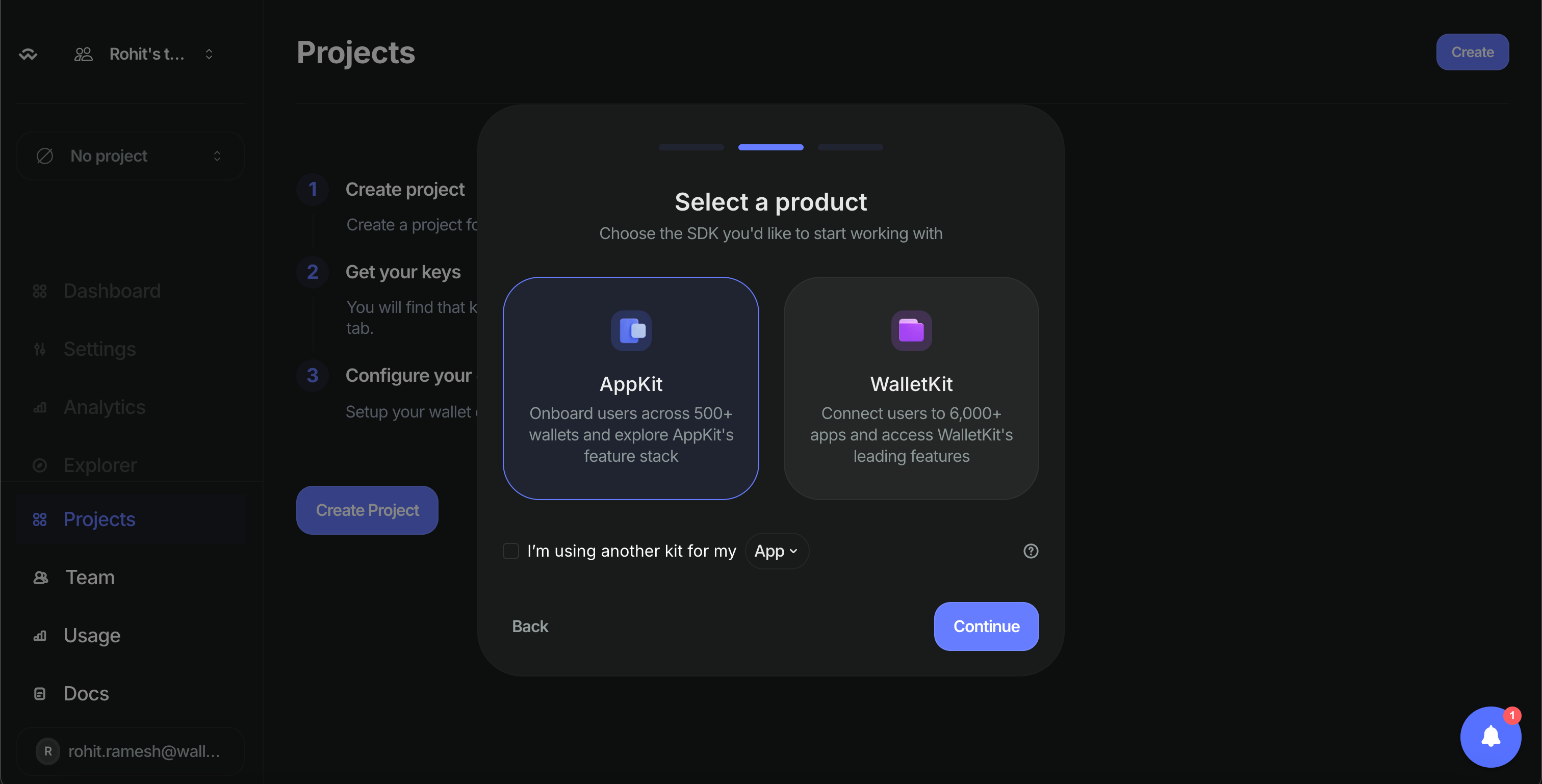
Select the framework as “Next.js” and click on “Create”. Reown Cloud will now create a new project for you which will also generate a project Id.
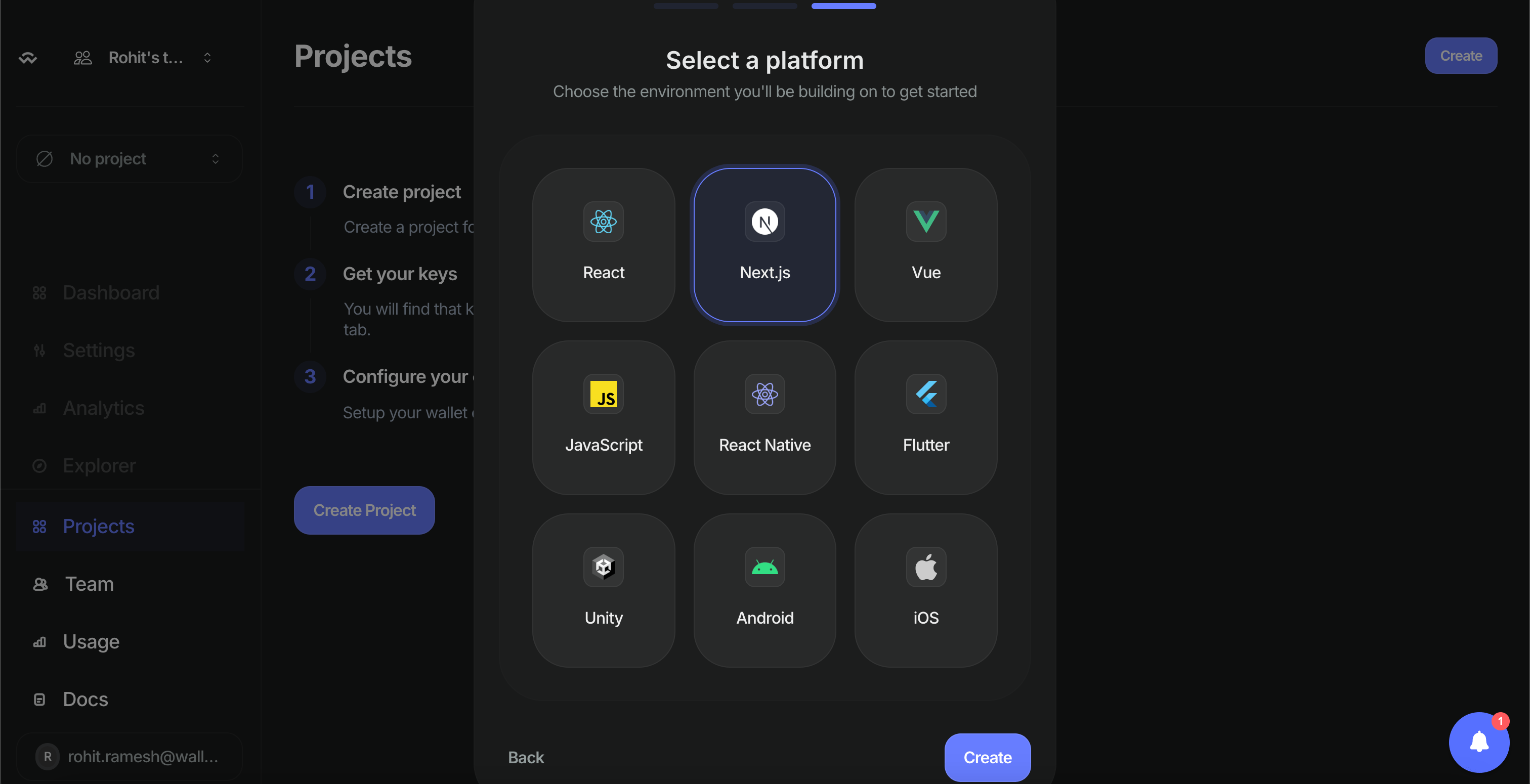
You will notice that your project was successfully created. On the top left corner, you will be able to find your Project Id. Please copy that as you will need that later.
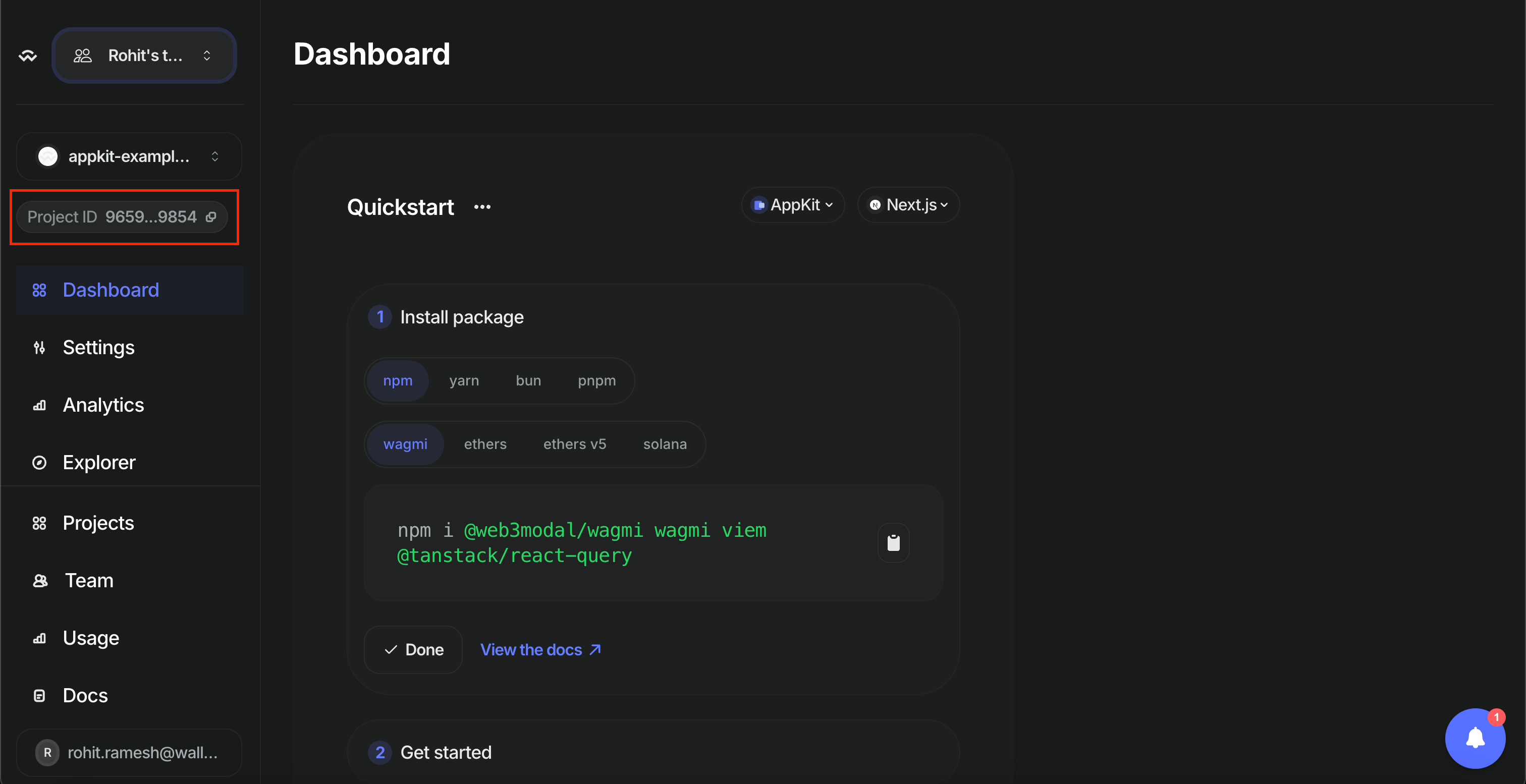
Build the App using AppKit
Before we build the app, let’s first configure our .env file. On the root level of your code directory, create a new file named .env.
Open that file and create a new variable NEXT_PUBLIC_PROJECT_ID. You will assign the project Id that you copied in the previous step to this environment variable that you just created. This is what it will look like:
NEXT_PUBLIC_PROJECT_ID = <YOUR_PROJECT_ID_HERE>
Note: Please make sure you follow the best practices when you are working with secret keys and other sensitive information. Environment variables that start with NEXT_PUBLIC will be exposed by your app which can be misused by bad actors.
Configure AppKit
On the root level of your code directory, create a new folder named config and within that folder, create a new code file named config/index.tsx. Now, paste the code snippet shared below inside the code file, i.e., config/index.tsx.
import { WagmiAdapter } from "@reown/appkit-adapter-wagmi";
import { cookieStorage, createStorage } from "wagmi";
import { mainnet, arbitrum } from '@reown/appkit/networks'
// Get projectId from https://cloud.reown.com
export const projectId = process.env.NEXT_PUBLIC_PROJECT_ID;
export const networks = [mainnet, arbitrum]
if (!projectId) throw new Error("Project ID is not defined");
// Set up the Wagmi Adapter (config)
export const wagmiAdapter = new WagmiAdapter({
storage: createStorage({
storage: cookieStorage
}),
ssr: true,
networks,
projectId
})
export const config = wagmiAdapter.wagmiConfig
So what's happening in the above code? Let's understand it step-by-step:
1. First, we need to import the necessary functions from their respective packages.
a. WagmiAdapter - this is used to create a WAGMI configuration which is then initialized to the wagmiAdapter
b. cookieStorage , createStorage - this provides a storage mechanism using cookies and a function to create custom storage solutions (in this case, using cookies).
Create the Modal for your app
Now, we need to create a context provider to wrap our application in and initialize AppKit.
On the root level of your code directory, create a new folder named context and within that folder, create a new code file named context/index.tsx. Now, paste the code snippet shared below inside the code file, i.e., context/index.tsx.
'use client'
import { wagmiAdapter, projectId } from '@/config'
import { createAppKit } from '@reown/appkit/react'
import { mainnet, arbitrum } from '@reown/appkit/networks'
import { QueryClient, QueryClientProvider } from '@tanstack/react-query'
import React, { type ReactNode } from 'react'
import { cookieToInitialState, WagmiProvider, type Config } from 'wagmi'
// Set up queryClient
const queryClient = new QueryClient()
if (!projectId) {
throw new Error('Project ID is not defined')
}
// Set up metadata
const metadata = { //this is optional
name: "appkit-example",
description: "AppKit Example - EVM",
url: "https://exampleapp.com", // origin must match your domain & subdomain
icons: ["https://avatars.githubusercontent.com/u/37784886"]
}
// Create the modal
const modal = createAppKit({
adapters: [wagmiAdapter],
projectId,
networks: [mainnet, arbitrum],
metadata: metadata,
features: {
analytics: true, // Optional - defaults to your Cloud configuration
email: true, // default to true
socials: ['google', 'x', 'github', 'discord', 'apple', 'facebook', 'farcaster'],
emailShowWallets: true, // default to true
},
themeMode: 'light'
})
function ContextProvider({ children, cookies }: { children: ReactNode; cookies: string | null }) {
const initialState = cookieToInitialState(wagmiAdapter.wagmiConfig as Config, cookies)
return (
<WagmiProvider config={wagmiAdapter.wagmiConfig as Config} initialState={initialState}>
<QueryClientProvider client={queryClient}>{children}</QueryClientProvider>
</WagmiProvider>
)
}
export default ContextProvider
Let’s understand what is happening in the above code:
1. First, we import the necessary functions from their respective packages. After this, we need to create the modal component for our app.
metadata - This object contains information about our application that will be used by AppKit. This includes the name of the app, the description, the url and the icons representing our app. This is optional.
createAppKit - this is called to initialize the AppKit component, which handles the user interface for connecting to blockchain wallets. The function is configured with various options, such as the app's metadata, theming, and enabling features like analytics and onramp services.
networks - these are the networks that we want our app to support. So import the chains you want your app to support from @reown/appkit/network and assign it to this network parameter. You can view the complete list of supported chains here.
socials - this configures the allowed social login methods for users to use to connect to your app.
WagmiProvider: Provides blockchain and wallet connection context to the app.
QueryClientProvider: Provides the React Query context for managing server-state data.
Now, let’s create the layout for our app. In app/layout.tsx , remove the existing code and paste the code snippet given below.
import type { Metadata } from "next";
import { Inter } from "next/font/google";
import "./globals.css";
const inter = Inter({ subsets: ["latin"] });
import { headers } from "next/headers"; // added
import ContextProvider from '@/context'
export const metadata: Metadata = {
title: "AppKit Example App",
description: "Powered by Reown"
};
export default function RootLayout({
children
}: Readonly<{
children: React.ReactNode
}>) {
const cookies = headers().get('cookie')
return (
<html lang="en">
<body className={inter.className}>
<ContextProvider cookies={cookies}>{children}</ContextProvider>
</body>
</html>
)
}
Create the UI for your app
For our app to have the UI with which your users can interact, you need to set a simple UI and configure the modal. Since, we have already set up AppKit, you can use <w3m-button> which will serve as a “Connect Wallet” button or you can build your own custom button using the hooks that AppKit provides.
Open the app/page.tsx file and remove the existing boilerplate code, and then replace it with the code snippet given below.
"use client";
import { useAccount } from "wagmi";
export default function Home() {
const { isConnected } = useAccount();
return (
<main className="min-h-screen px-8 py-0 pb-12 flex-1 flex flex-col items-center">
<header className="w-full py-4 flex justify-between items-center">
<div className="flex items-center">
<img src="/walletconnect.png" alt="logo" className="w-10 h-10 mr-2" />
<div className="hidden sm:inline text-xl font-bold">Reown - AppKit + EVM</div>
</div>
</header>
<h2 className="my-8 text-2xl font-bold leading-snug text-center">Examples</h2>
<div className="max-w-4xl">
<div className="grid bg-white border border-gray-200 rounded-lg overflow-hidden shadow-sm">
<h3 className="text-sm font-semibold bg-gray-100 p-2 text-center">Connect your wallet</h3>
<div className="flex justify-center items-center p-4">
<w3m-button />
</div>
</div>
<br></br>
{isConnected && (
<div className="grid bg-white border border-gray-200 rounded-lg overflow-hidden shadow-sm">
<h3 className="text-sm font-semibold bg-gray-100 p-2 text-center">Network selection button</h3>
<div className="flex justify-center items-center p-4">
<w3m-network-button />
</div>
</div>
)}
</div>
</main>
);
}
The code above uses the AppKit configuration to provide two buttons: one for users to connect their wallet to the app, and another to allow users to switch networks.
You can now run the app and test it out. In order to do so, run the command given below.
npm run dev
If you are using alternative package managers, you can try either of these commands - yarn dev, or pnpm dev, or bun dev.
Conclusion
And that’s it! You have now learned how to create a simple app using AppKit that allows users to connect their wallet and interact with any EVM network. Reown AppKit is a powerful solution for developers looking to integrate wallet connections and other Web3 functionalities into their apps on any EVM chain. In just a few simple steps, you can provide your users with seamless wallet access, one-click authentication, social logins, and notifications—streamlining their experience while enabling advanced features like on-ramp functionality and smart accounts. By following this guide, you'll quickly get up and running with Reown’s AppKit, enhancing your app’s user experience and interaction with blockchain technology.
You can view the complete code repository here.
What's Next?
If you're wondering how to use Reown for various use cases and build apps with great UX, feel free to check out our other blogs here.
Need help?
For support, please join the official Reown Discord Server.